Hello,
Are you new to SharePoint or do you just want to learn how to develop SharePoint modern solutions? Then, you came to the right place!
This is a guide to help you learn SharePoint Framework (SPFx), a framework to develop SharePoint (and Teams) client-side solutions. SPFx leverages the following technologies:
The Basics
SharePoint Framework is a page and web part model that provides full support for client-side SharePoint development, easy integration with SharePoint data, and extending Microsoft Teams. With the SharePoint Framework, you can use modern web technologies and tools in your preferred development environment to build productive experiences and apps that are responsive and mobile-ready.
The SPFx is the recommended SharePoint customization and extensibility model for developers. Due to tight integration between SharePoint Online, Microsoft Teams, and Microsoft Viva Connections, developers can also use SPFx to customize and extend all these products. In fact, SPFx is the only extensibility and customization option for Viva Connections.
In addition to SharePoint Online, SPFx is supported and can be used to customize SharePoint on-premises deployments going back to SharePoint Server 2016.
With the SharePoint Framework, we can develop:
Other useful links:
- Overview of the SharePoint Framework (SPFx)
- Scaffold projects by using Yeoman SharePoint generator
- SharePoint Framework development tools and libraries
- SharePoint Framework toolchain
Technology stack
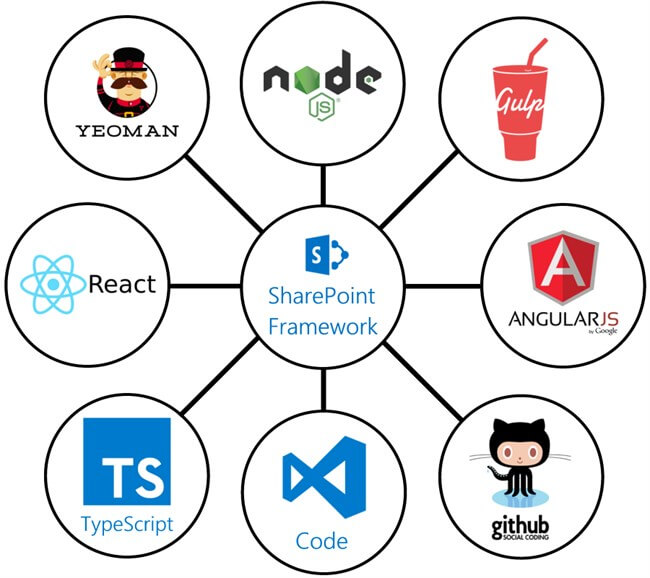
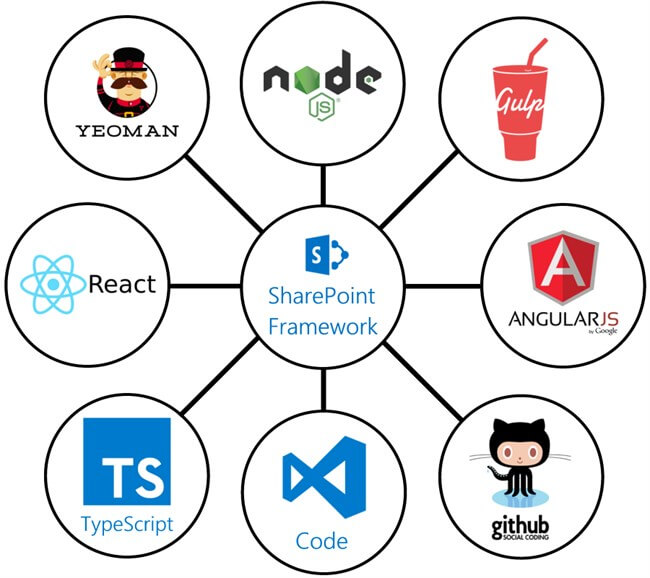
SPFx Development Toolchain
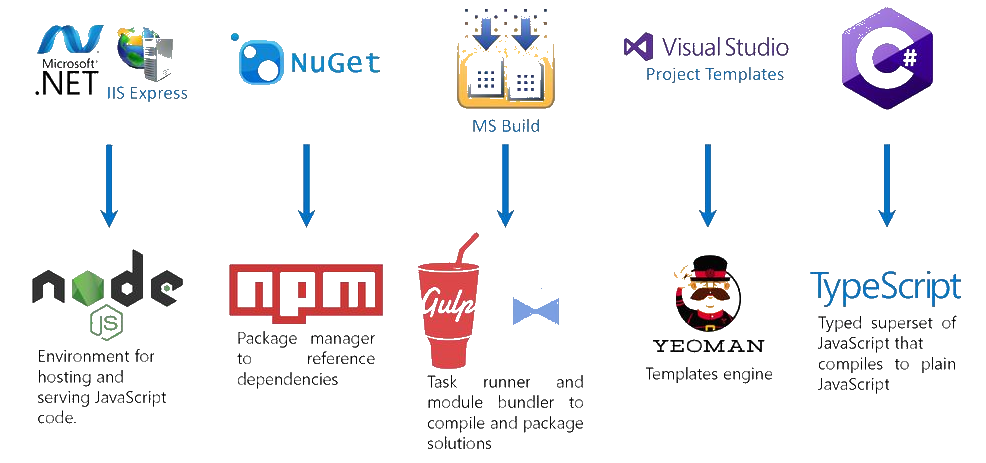
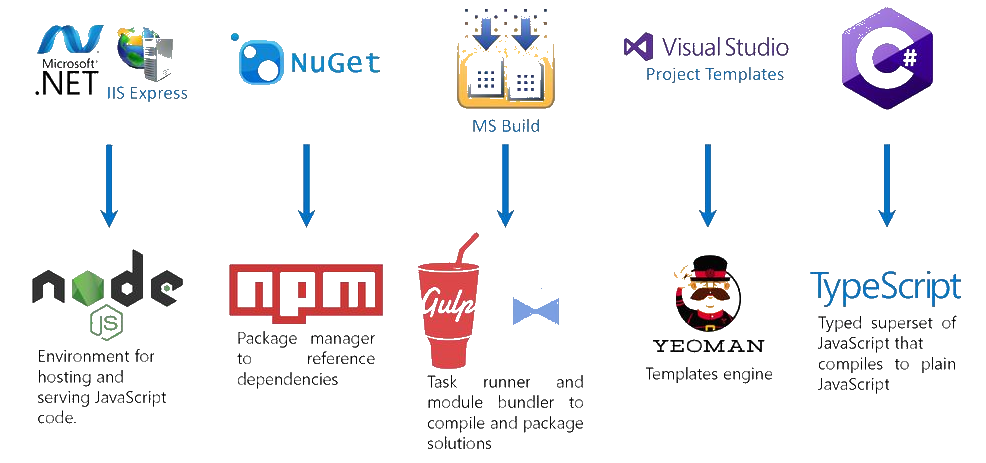
TypeScript
TypeScript is the preferred programming language to develop in SPFx. TypeScript is a programming language and maintained by Microsoft and is a superset of JavaScript and adds optional static typing to the language to develop client-side solutions. It is designed for the development of large applications and transpiles to JavaScript to be executed in the browser.
Useful TypeScript concepts:
- const – allows the definition of a variable whose value is not going to be changed. Use this instead of let if the variable value is not going to be changed after its declaration.
- let – allows the definition of a variable whose value is going to be changed. Use this instead of const if the variable value is going to be changed after its declaration.
- var – allows the definition of a variable whose value is going to be changed. To be avoided since variables declared with var are acessible from any scope (scope (function, module, namespace, global)
- type – allows the definition of a data class without any business logic or methods, just data. It can be also useful to avoid the usage of magic strings in the code.
- enum – allows the definition of a enumeration
- interface – allows the definition of a interface that can then be implemented by a concret class.
- class – allows the definition of a class that optionally implements an interface
- extends – when used, it allows a class to inherit from another class
- export – allows the export of functions, variables, interfaces and classes that can be imported with the import statement
- import – allows the import of functions, variables, interfaces and classes exported with the export statement
Useful links:
- TypeScript – The Basics (video)
- The starting point for learning TypeScript
- TypeScript for the New Programmer
- TypeScript Tutorials
- Clean Code concepts adapted for TypeScript
React
React is a JavaScript library for building user interfaces and is at the core of SPFx to build advanced applications.
- Declarative: React makes it painless to create interactive UIs. Design simple views for each state in your application and React will efficiently update and render just the right components when your data changes. Declarative views make your code more predictable, simpler to understand, and easier to debug.
- Component-Based: Build encapsulated components that manage their state, then compose them to make complex UIs. Since component logic is written in JavaScript instead of templates, you can easily pass rich data through your app and keep the state out of the DOM.
- Learn Once, Write Anywhere: We don’t make assumptions about the rest of your technology stack, so you can develop new features in React without rewriting existing code. React can also render on the server using Node and power mobile apps using React Native.
Useful links:
- React Official Web Site
- Tutorial: Intro to React
- React Component LifeCycle
- How to get started with React for building advanced SPFx solutions
- SharePoint Online: CRUD operations using SPFx ReactJS framework
- How to React ⚛️
- Fluent UI (Microsoft Components for Microsoft 365 Applications)
- React: Lists and Keys
- How to Implement Memoization in React to Improve Performance
To help developers to build advanced SPFx solutions, there are a lot of React controls, either developed by Microsoft such as Fluent UI or by communities such as the PnP community.
PnP JS
PnP JS is a NPM package that simplifies interaction with SharePoint Online and Microsoft Graph.
Useful links:
- Working with SharePoint List Items
- Use @pnp/sp (PnPJS) library with SharePoint Framework web parts
- A Complete Guide to Getting and Setting Fields Value using PnP JS in SPFx
- Using PnP JS with Microsoft Graph
- PnP SDKs
Repositories
Here are some of the most useful repositories to start learning SharePoint Framework:
- Microsoft 365 Community
- Microsoft 365 Community Repositories
- SharePoint Framework web part, Teams tab, personal app, app page samples
- Reusable React controls for SPFx solutions
- sp-dev-fx-webparts
- pnp/sp-dev-fx-extensions
- pnp/sp-dev-fx-property-controls
- pnp/sp-dev-fx-aces
- Microsoft 365 Unified Sample Gallery with more than 1000 samples
Concepts / Tooling
Core SPFx Concepts
NPM Packages
SharePoint client-side development tools use the npm package manager, like NuGet, to manage dependencies and other required JavaScript helpers. npm is typically included as part of Node.js setup.
Package installation modes:
- npm install @package – installs the package
- npm install @package –save – installs the package and updates package.json automatically (dependencies section)
- npm install @package@version –save-dev – installs the package and updates package.json automatically (devDependencies section)
- npm install @package@version –save – installs the package with the specified version and updates package.json automatically (dependencies section)
- npm install @package@version –save-dev – installs the package with the specified version and updates package.json automatically (devDependencies section)
Useful npm packages to include in a SPFx solution:
- npm install gulp yo @microsoft/generator-sharepoint@<spfx_version> –global
- Example: npm install gulp yo @microsoft/generator-sharepoint@1.15.2 –global
- npm install @microsoft/microsoft-graph-types –save
- npm install @pnp/sp –save
- npm install @pnp/graph –save
- npm install @fluentui/react –save
- npm install @pnp/spfx-controls-react –save
- npm install @pnp/spfx-property-controls –save
- npm install localforage –save
- npm install @luudjanssen/localforage-cache –save
- npm install spfx-fast-serve -g
- npm install -g pnpm
Gulp Commands
- gulp clean – cleans the solution. Cleans the dist and temp folders from the solution
- gulp trust-dev-cert – installs the development certificate
- gulp build – builds the solution, tranforms TypeScript into JavaScript
- gulp bundle – bundles the solution and minimizes CSS and JS (in debug mode)
- gulp bundle –ship – bundles the solution and minimizes CSS and JS for deployment in SharePoint in production mode (release mode)
- gulp package-solution – generates package (.sppkg file) but to run in debug mode
- gulp package-solution –ship – generates package (.sppkg file) to run in production mode
- gulp serve – launches SharePoint Workbench to test web parts in debug mode
- gulp serve –nobrowser – allows testing web parts in debug mode but without launching SharePoint Workbench
SPFx Development Environment
Setup Development Environment
To setup your development environment, run the following commands from the command prompt:
- npm install gulp-cli yo @microsoft/generator-sharepoint –global
- npm install webpack –global
- npm install –save-dev webpack
- gulp trust-dev-cert
Node Version Manager (nvm)
Node Version Manager (NVM) allows developers to install multiple versions of node. This can be useful when working with different SPFx versions that use different versions of Node.
To download NVM, click here.
Install Node using NVM
After installing NVM, to install a node version use the following command:
nvm install <node_version>. Example: nvm install 14.19.1
Switch between versions of Node
If you have multiple versions of Node, you can switch between them using the following command:
nvm use <node_version>. Example: nvm use 12.22.10
To have a full list of the installed node versions, run “nvm list”.
SPFx Fast Serve
If you have a big SPFx solution, gulp serve can take a lot of time, especially if you want to rapidly test your developments. SPFx Fast Serve is a command line utility, which modifies your SharePoint Framework solution, so that it runs continuous serve command as fast as possible. To know more, click here.
Here is a comparison between gulp serve and SPFx Fast Serve (times depend on hardware, environment). The times listed below are “refresh” time (the time needed to compile your project when you change a file and start refreshing a page in a browser):
gulp serve | spfx-fast-serve | |
---|---|---|
Default “Hello World” React web part | 3-5 sec | 0.1-0.2 sec |
PnP Modern Search solution | 28-34 sec | 2-4 sec |
SP Starter Kit solution (v1) | 40-50 sec | 2-3 sec |
This is a must have for every SharePoint Framework Developer!
Recommended Visual Studio Code Extensions
- Auto Rename Tag
- Tabnine
- VSCode icons
- Bracket Pair Colorizer
- Visual Code IntelliCode
- SonarLint for Visual Studio Code
- PowerShell
- Live Server
- Glean
- Thunder Client
- SPFx Snippets
- GitLens
- Todo Tree
- ESLint
SPFx Quick Start
The most common development component in SPFx is the web part. To better understand how to create your first web part, read this article. In SPFx client-side web parts, we have the following components:
- Web part main component – acts as a container to the React component that has the main logic. In this example, we have a web part class called BirthdaysWorkAnniversariesNewCollaboratorsWebPart that renders the BirthdaysWorkAnniversariesNewCollaborators React component that has the web part main logic.
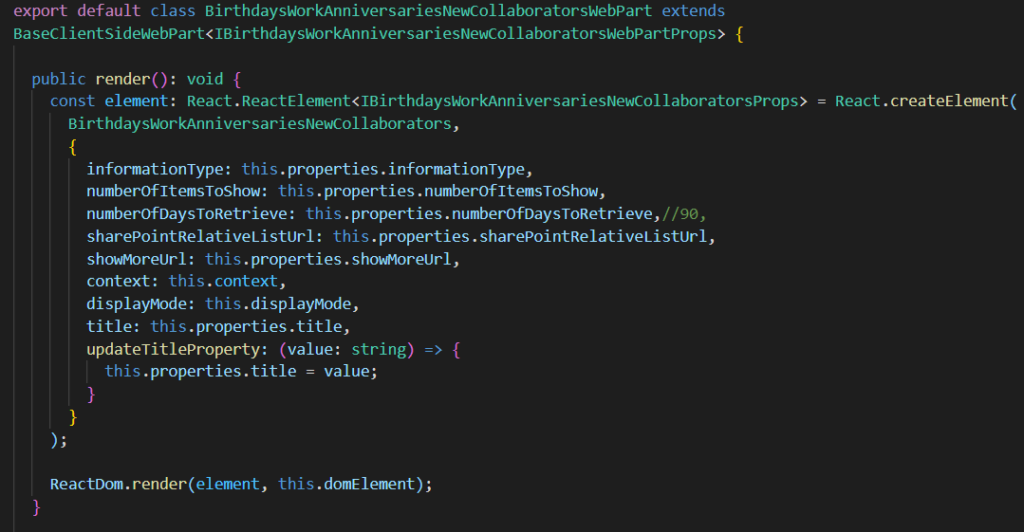
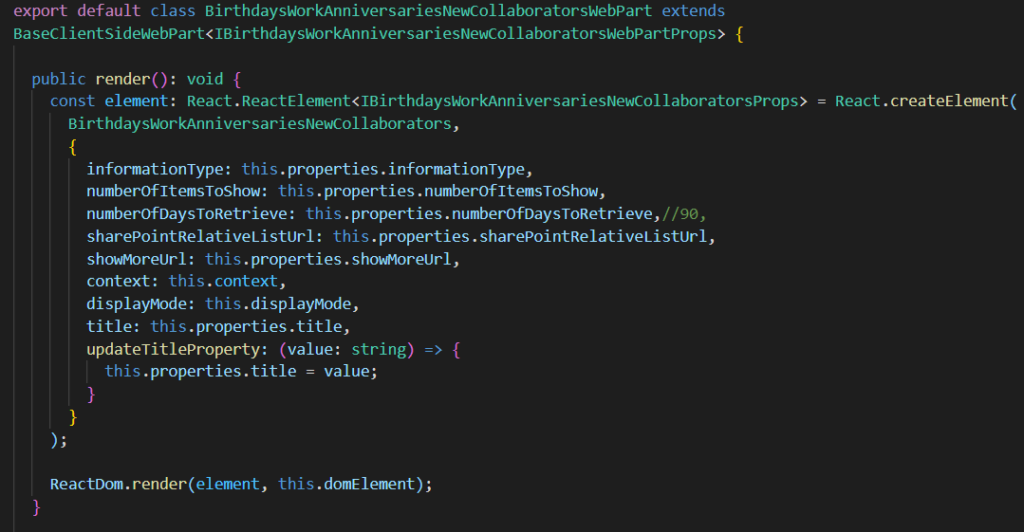
- React component associated with the web part. This file has a render method responsible for the rendering of the web part UI and uses two major React concepts: props supplied by the web part that allows users to configure the web part behavior and state that also allows to control how the the web part is rendered. The main difference between props and state is that state can be changed and props can’t (in the code they are readonly). In the example below, we have the BirthdaysWorkAnniversariesNewCollaborators React component that was called by the BirthdaysWorkAnniversariesNewCollaboratorsWebPart web part.
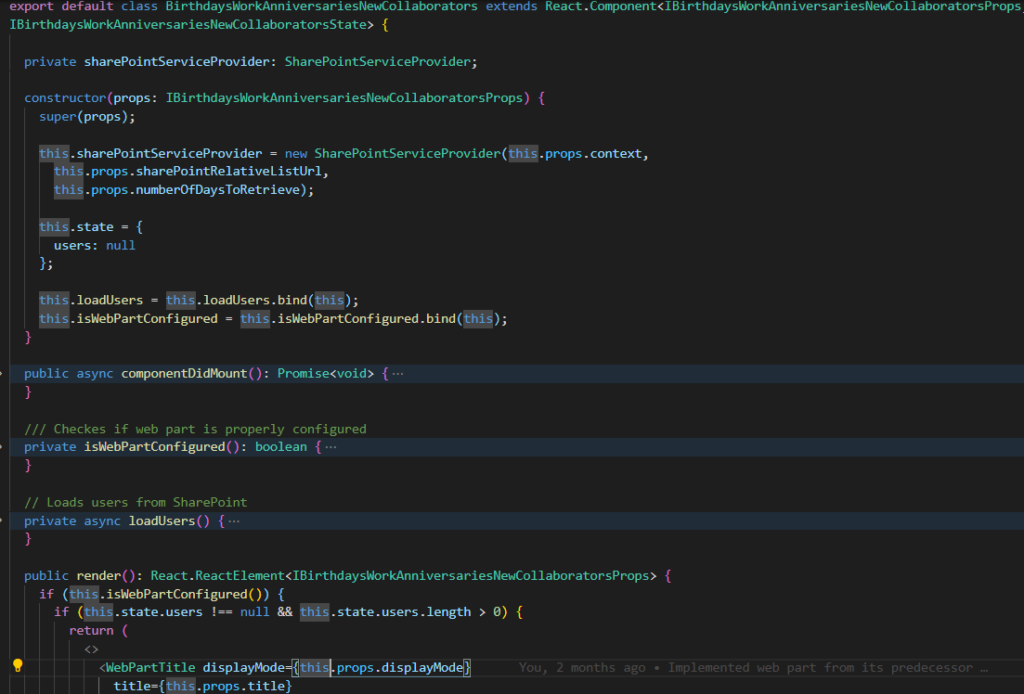
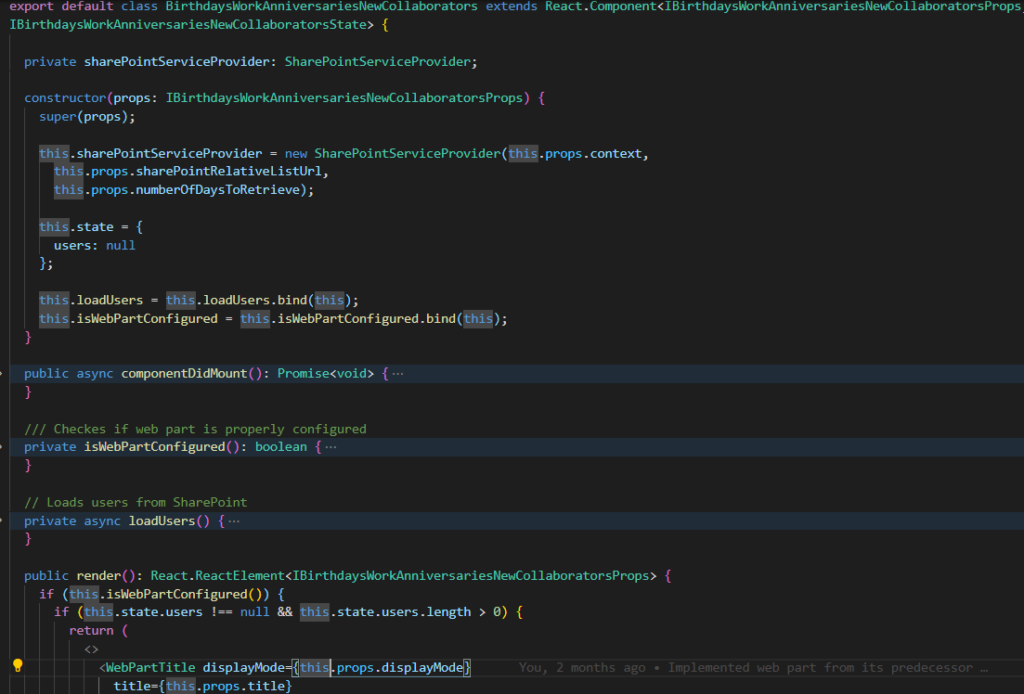
- Other associated files such as:
- Service classes to get data from SharePoint or Microsoft Graph for instance
- Data object classes (models)
- Helper classes
- etc
SPFx Tutorials
Getting Started with SPFx
- SharePoint Framework Tutorial 1 – HelloWorld WebPart
- SharePoint Framework Tutorial 2 – HelloWorld, Talking to SharePoint
- SharePoint Framework Tutorial 3 – HelloWorld, Serving in SharePoint Page
- SharePoint Framework Tutorial 4 – HelloWorld, Deploy to Office 365 CDN
- SharePoint Framework Tutorial – Using Microsoft Graph APIs
- SharePoint Framework Tutorial – Surfacing your solution as a Microsoft Teams tab
- SharePoint Framework Tutorial – Using Office UI Fabric React Components
- SharePoint Framework Tutorial – Provisioning SharePoint assets from SPFx solution
- SharePoint Framework Tutorial – Create a Full Width Web Part Page with SPFx
- Debugging SharePoint Framework solutions in Visual Studio Code
- Work with SharePoint Content using the SharePoint Framework
Developing SPFx Extensions
- SharePoint Framework Extensions Tutorial 1 – Build your first Extension
- SharePoint Framework Extensions Tutorial 2 – Using page placeholders from Application Customizer
- SharePoint Framework Extensions Tutorial 3 – Deploy your extension to site collection
- SharePoint Framework Extensions Tutorial 4 – Hosting extension from Office 365 CDN
- SharePoint Framework Extensions Tutorial – Build your first Field Customizer
- SharePoint Framework Extensions Tutorial – Build your first ListView Command Set
SharePoint CRUD Operations with SPFx
- SharePoint List Items CRUD Operations Demo Using PnPJS In React based SPFx Webpart
- SharePoint CRUD Sample with Details List Fluent UI Control and a SharePoint List
Graph API
Related Articles
To learn why your business should migrate to SharePoint Online and Office 365, click here and here.
If you want to learn how you can rename a modern SharePoint site, click here.
If you want to learn how to save time time scheduling your meetings, click here.
If you want to learn how to enable Microsoft Teams Attendance List Download, click here.
If you want to learn how to create a dynamic org-wide team in Microsoft Teams with all active employees, click here.
If you want to modernize your SharePoint classic root site to a modern SharePoint site, click here.
If you are a SharePoint administrator or a SharePoint developer who wants to learn more about how to install a SharePoint 2019 farm in an automated way using PowerShell, I invite you to click here and here.
If you learn how to greatly speed up your SharePoint farm update process to ensure your SharePoint farm keeps updated and you stay one step closer to start your move to the cloud, click here.
If you prefer to use the traditional method to update your farm and want to learn all the steps and precautions necessary to successfully keep your SharePoint farm updated, click here.
If you want to learn how to upgrade a SharePoint 2013 farm to SharePoint 2019, click here and here.
If SharePoint 2019 is still not an option, you can learn more about how to install a SharePoint 2016 farm in an automated way using PowerShell, click here and here.
If you want to learn how to upgrade a SharePoint 2010 farm to SharePoint 2016, click here and here.
If you are new to SharePoint and Office 365 and want to learn all about it, take a look at these learning resources.
If you are work in a large organization who is using Office 365 or thinking to move to Office 365 and is considering between a single or multiple Office 365 tenants, I invite you to read this article.
If you want to know all about the latest SharePoint and Office 365 announcements from Ignite and some more recent announcements, including Microsoft Search, What’s New to Build a Modern Intranet with SharePoint in Office 365, Deeper Integration between Microsoft Teams and SharePoint and the latest news on SharePoint development, click here.
If your organization is still not ready to go all in to SharePoint Online and Office 365, a hybrid scenario may be the best choice. SharePoint 2019 RTM was recently announced and if you to learn all about SharePoint 2019 and all its features, click here.
Happy SharePointing!